Dynamic Programming in Python: Top 10 Problems (with Code)
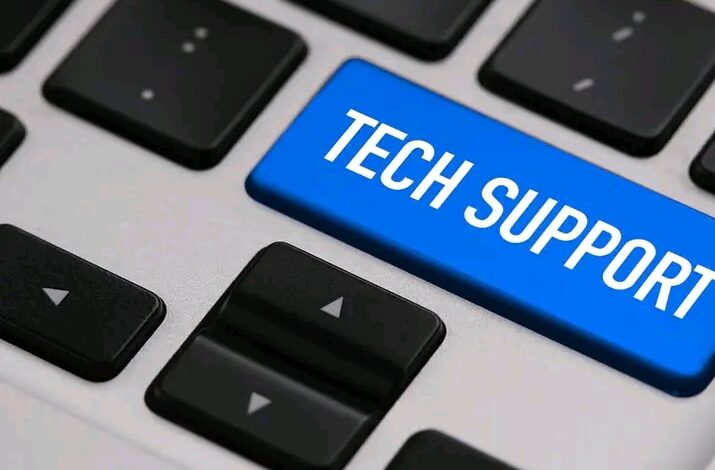
Dynamic programming is a powerful algorithmic technique used in computer science and mathematics to solve optimization problems by breaking them down into smaller, overlapping subproblems.
It is particularly useful when the problem exhibits overlapping substructures, allowing us to solve each subproblem only once and store its solution for future use.
In this article, we will explore dynamic programming in Python and present the top 10 problems along with their corresponding code implementations.
What is Meant by Dynamic Programming?
Dynamic programming refers to a methodical approach to problem-solving where a complex problem is divided into smaller, simpler subproblems.
By solving these subproblems and storing their solutions, we can avoid redundant calculations and improve the overall efficiency of the solution.
This technique is especially useful when the problem can be divided into overlapping subproblems that can be solved independently.
What is Dynamic Programming with an Example?
To illustrate dynamic programming, let’s consider the classic example of the Fibonacci sequence.
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones: 0, 1, 1, 2, 3, 5, 8, 13, 21, and so on.
Using dynamic programming, we can efficiently calculate the nth Fibonacci number by breaking it down into smaller subproblems.
Here’s an example Python code snippet that demonstrates this approach:
“`python
def fibonacci(n):
if n <= 1:
return n
else:
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i – 1] + fib[i – 2]
return fib[n]
“`
In this code, we use a list `fib` to store the solutions to the subproblems.
By iteratively calculating the Fibonacci numbers up to `n`, we avoid redundant calculations and achieve a more efficient solution.
What is a Real Example of Dynamic Programming?
One real-world example of dynamic programming is the knapsack problem.
Suppose you are a burglar and you want to maximize the value of the items you can steal from a house. However, you can only carry a limited weight in your knapsack.
Each item has a certain value and weight associated with it. The goal is to determine the combination of items that maximizes the total value while staying within the weight limit.
This problem can be efficiently solved using dynamic programming, where the subproblems correspond to different weight limits and subsets of the items.
What Uses Dynamic Programming?
Dynamic programming finds applications in various domains, including:
1. Algorithm design
Dynamic programming provides efficient solutions for a wide range of problems, such as shortest paths, sequence alignment, and matrix chain multiplication.
2. Economics and finance
Dynamic programming techniques are used to optimize resource allocation, portfolio management, and investment strategies.
3. Operations research
Dynamic programming helps in solving optimization problems related to resource allocation, production planning, and scheduling.
4. Artificial intelligence
Dynamic programming is employed in areas such as reinforcement learning, robotics, and natural language processing to solve complex decision-making problems.
FAQ
What is the difference between dynamic programming and divide-and-conquer?
Dynamic programming breaks down a problem into overlapping subproblems and solves each subproblem only once, while divide-and-conquer divides a problem into non-overlapping subproblems that are solved independently.
Is dynamic programming applicable to all types of problems?
No, dynamic programming is most effective when the problem exhibits overlapping substructures and can be divided into smaller subproblems.
How do I identify if a problem can be solved using dynamic programming?
Look for the following characteristics: overlapping subproblems, optimal substructure, and the possibility of breaking down the problem into smaller, independent subproblems.
Are there any drawbacks or limitations to dynamic programming?
Dynamic programming can have high memory requirements, especially for problems with large input sizes. Additionally, some problems may not have the required optimal substructure for dynamic programming to be applicable.